
Freeze Authority Code in a Solana Smart Contract
Scenario
Picture this: you just bought into a promising new token. The project has a nice-looking website and an active community. The chart is climbing quickly. You’re feeling good. You’re thinking, maybe this is finally the one? Then, out of nowhere, trading stops. You can’t sell. You can’t do anything. But some wallets seem to be moving just fine. You can actively see them selling in the order book. You sit back helplessly and watch your gains disappear in one giant red candle.
What Happened?
These wallets are draining liquidity and leaving everyone else stuck with worthless tokens. It’s not a glitch or bad luck. This is done on purpose by the developers. It was their plan all along, and they tricked you with some sneaky code written into the token’s smart contract.
Freeze Authority
This sneaky code is known as “Freeze Authority” on Solana and as “Pausable” (or similar) on EVM blockchains. On EVM chains, developers can also stop trading by including specific functions in the code to whitelist or blacklist particular wallets. This prevents you from selling while their wallets are whitelisted for trading.
How to Check for Freeze Authority
Just grab the contract address and enter it into a rug checker website. These checks can be easily done on sites like Solsniffer and Quill Ai. You can easily find a rug checker for your blockchain of choice by googling something like “rug check Base chain” or “rug check PulseChain.” See the following images for an example:
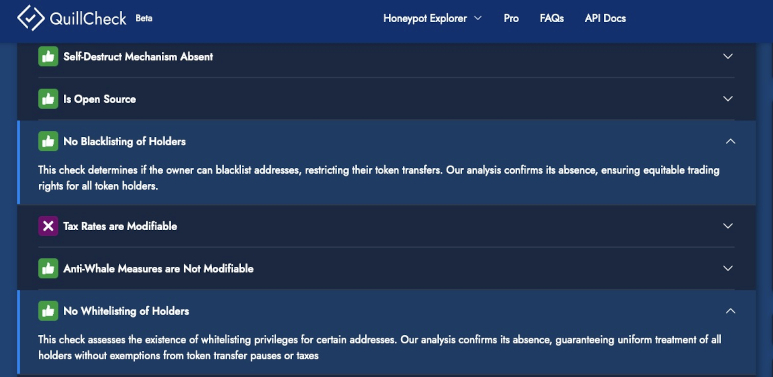
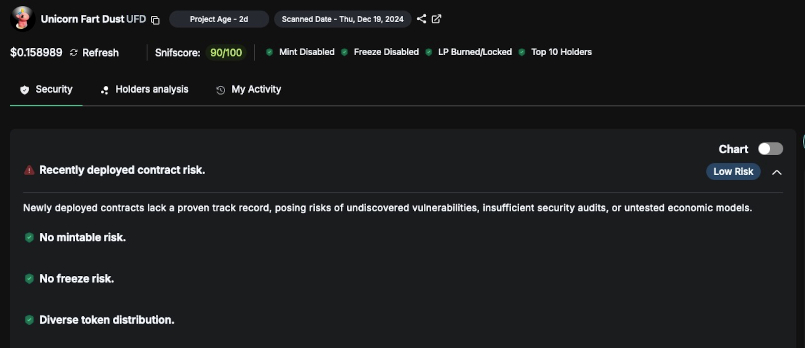
This is a simple check that you should always do before buying any type of crypto on a DEX.
Note: You may see freeze authority or the ability to pause trading on some larger projects, as this was intended as a fail-safe for hackers or any various problems within a cryptocurrency. This does not always mean danger, but you shouldn’t buy meme coins and similar tokens on a DEX with a freezable contract—or you will get rugged.
Also note: Just because there’s not a freeze authority enabled doesn’t mean your coin is safe. There are many scams that don’t have this feature. This is just one of many things you should be checking.
The Mechanics of How Tokens Are Frozen – Ethereum Chain
Note: If you don’t want to dive deep into code, you can skip the rest of this article. You’ll be fine just using a rug-check website.
On the Ethereum blockchain and EVM-like chains, tokens can be frozen if the smart contract includes specific functions that allow the creator to restrict transfers. They usually use the “Pausable” feature from libraries like OpenZeppelin. Here’s what it may look like:
Solidity
function pause() public onlyOwner {
_pause();
}
function unpause() public onlyOwner {
_unpause();
}
function _beforeTokenTransfer(address from, address to, uint256 amount) internal whenNotPaused {
super._beforeTokenTransfer(from, to, amount);
}
Blacklisting
Developers use blacklisting to stop specific addresses from transferring or receiving tokens. Let’s say a whale buys into a token, and the dev wants to prevent them from selling. They could add the whale’s wallet address into the blacklist function. Here’s an example coded in Solidity:
mapping(address => bool) private _blacklist;
function blacklist(address account) public onlyOwner {
_blacklist[account] = true;
}
function removeFromBlacklist(address account) public onlyOwner {
_blacklist[account] = false;
}
function _beforeTokenTransfer(address from, address to, uint256 amount) internal {
require(!_blacklist[from], "Sender is blacklisted");
require(!_blacklist[to], "Recipient is blacklisted");
super._beforeTokenTransfer(from, to, amount);
}
If you want to get nerdy and do some deep digging, you can look through the code yourself. Some more functions you can look for are: pause
, unpause
, blacklist
, freeze
, or _beforeTokenTransfer.
Modifiers: Search for terms like whenNotPaused
or conditions using mappings like _blacklist.
Libraries: Check if the contract imports libraries such as OpenZeppelin’s Pausable.
How Freeze Authority Code Looks on the Solana Blockchain
The code isn’t embedded directly in the token’s custom program but is part of the Solana Token Program library. The token creator specifies the freeze authority when creating the token mint. Below is an example of Rust code for creating a token with freeze authority:
let freeze_authority = Some(admin_pubkey);
let token_mint = spl_token::instruction::initialize_mint(
&spl_token::id(),
&mint_pubkey,
&mint_authority_pubkey,
freeze_authority.as_ref(),
decimals,
)?;
The key part here is the freeze_authority
parameter. If this is None
, no one can freeze accounts.
Example Rust code for freezing a token account:
let instruction = spl_token::instruction::freeze_account(
&spl_token::id(),
&token_account_pubkey,
&mint_pubkey,
&freeze_authority_pubkey,
)?;
If the Freeze Authority field is populated, it means someone has the ability to freeze accounts. If Freeze Authority is set to None
, no one can freeze accounts, which is generally safer for investors.
Summary
Before buying any coins on a DEX, check that the coin can’t be frozen. This is easily done by using various rug checker websites.
S Taylor is a crypto trader with five years of experience, having navigated a wide range of market dynamics and witnessed numerous scams firsthand. As a former victim of scams, S Taylor turned their focus to blockchain forensics and Solidity Smart Contract development, gaining deep technical expertise in the field. With a unique insider’s perspective, they’ve been involved in various crypto projects, where they’ve seen how developers can exploit vulnerable investors.
S Taylor is also the published author of Meme Coins Made Easy, a comprehensive guide that teaches beginners about cryptocurrency and how to identify and avoid common scams. S Taylor is dedicated to sharing valuable insights and helping the crypto community stay informed and safe.
Disclaimer: This article is for informational purposes only and should not be considered legal, tax, investment, or financial advice.